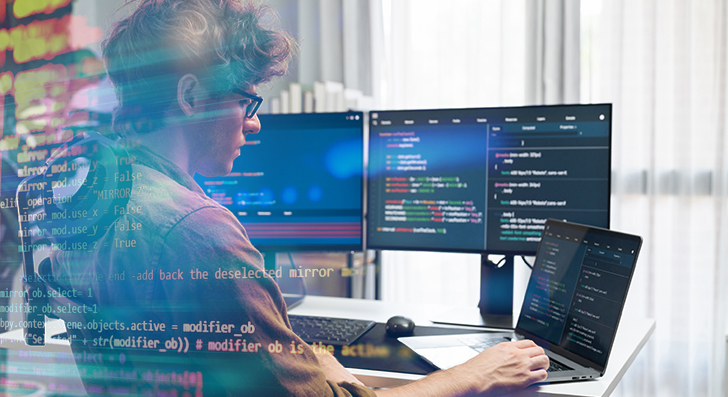
Scalability usually means your application can handle advancement—additional end users, a lot more data, and more targeted visitors—devoid of breaking. Being a developer, developing with scalability in mind saves time and strain later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is not a little something you bolt on later on—it ought to be element of your prepare from the beginning. A lot of applications are unsuccessful after they mature quickly because the initial design can’t take care of the additional load. Like a developer, you should Imagine early about how your process will behave under pressure.
Commence by building your architecture for being adaptable. Stay away from monolithic codebases the place every thing is tightly linked. Instead, use modular layout or microservices. These patterns break your application into smaller sized, unbiased areas. Just about every module or services can scale By itself without impacting The complete program.
Also, give thought to your database from day one particular. Will it have to have to deal with 1,000,000 people or just a hundred? Pick the right variety—relational or NoSQL—based upon how your information will increase. System for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another significant point is to prevent hardcoding assumptions. Don’t create code that only will work less than present-day circumstances. Consider what would come about If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like information queues or celebration-driven techniques. These support your app manage additional requests devoid of finding overloaded.
If you Create with scalability in mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future problems. A very well-prepared program is easier to take care of, adapt, and improve. It’s greater to get ready early than to rebuild later.
Use the proper Database
Choosing the suitable database is really a key Component of constructing scalable programs. Not all databases are built the exact same, and using the Mistaken one can gradual you down and even cause failures as your application grows.
Commence by understanding your details. Could it be highly structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely sturdy with relationships, transactions, and regularity. They also assist scaling tactics like study replicas, indexing, and partitioning to manage much more targeted visitors and info.
In the event your knowledge is more versatile—like person action logs, item catalogs, or paperwork—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally much more simply.
Also, consider your read through and create designs. Are you carrying out many reads with fewer writes? Use caching and browse replicas. Will you be managing a hefty publish load? Take a look at databases which will tackle higher publish throughput, or maybe event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Imagine ahead. You may not want Innovative scaling options now, but choosing a database that supports them indicates you gained’t need to have to change afterwards.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And constantly watch databases effectiveness when you improve.
In short, the right database depends on your application’s composition, velocity desires, And just how you assume it to increase. Just take time to choose correctly—it’ll preserve plenty of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, each and every tiny delay provides up. Inadequately penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop economical logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away nearly anything unneeded. Don’t choose the most elaborate Option if an easy one will work. Maintain your capabilities small, targeted, and easy to check. Use profiling equipment to locate bottlenecks—sites the place your code requires much too prolonged to run or works by using a lot of memory.
Next, have a look at your database queries. These often sluggish things down in excess of the code itself. Ensure that Every question only asks for the data you really have to have. Keep away from SELECT *, which fetches almost everything, and as an alternative find particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout large tables.
In case you notice the identical facts becoming asked for again and again, use caching. Retailer the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations whenever you can. In place of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with massive datasets. Code and queries that get the job done fine with 100 records may well crash whenever they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your software keep sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more customers and much more site visitors. If every little thing goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. Both of these instruments support maintain your app quick, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout many servers. As an alternative to one particular server carrying out each of the function, the load balancer routes users to various servers according to availability. This suggests no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it may be reused rapidly. When users ask for the identical information yet again—like a product web site or possibly a profile—you don’t must fetch it within the databases every time. You may serve it within the cache.
There's two frequent types of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for rapid access.
two. Consumer-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching reduces databases load, improves velocity, and tends to make your application more productive.
Use caching for things which don’t modify normally. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Collectively, they assist your app manage extra customers, keep speedy, and recover from difficulties. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable programs, you require equipment that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really have to buy hardware or guess long term capability. When site visitors will increase, it is possible to insert extra assets with just a couple clicks or routinely working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also offer you services like managed databases, storage, load balancing, and security applications. You can focus on setting up your application in place of controlling infrastructure.
Containers are One more crucial Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person device. This can make it effortless to move your application involving environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of multiple containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If just one element of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for functionality and reliability.
In a nutshell, utilizing cloud and container instruments indicates you could scale quickly, deploy conveniently, and Get better rapidly when complications take place. If you prefer your app to improve with out boundaries, start working with these tools early. They preserve time, reduce threat, and assist you remain centered on setting up, not fixing.
Keep an eye on Everything
Should you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your app is executing, location challenges early, and make much better choices as your application grows. It’s a vital A part of creating scalable devices.
Get started by tracking fundamental metrics like CPU utilization, memory, disk Room, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Keep an eye on how long it takes for customers to load pages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place within your code.
Build alerts for significant challenges. Such as, In the event your reaction time goes earlier mentioned a Restrict or even a support goes down, you ought to get notified right away. This assists you repair problems fast, normally right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a different attribute and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and details enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate equipment set up, you keep in control.
Briefly, monitoring can help you keep your app trusted and scalable. It’s not nearly recognizing failures—it’s about comprehending your procedure and ensuring it really more info works effectively, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest apps need to have a solid foundation. By coming up with cautiously, optimizing correctly, and using the appropriate applications, you'll be able to Establish apps that increase smoothly devoid of breaking under pressure. Commence compact, Believe massive, and build wise.